Create Your own MFC Dialog Project with a EDIT control and 4 buttons
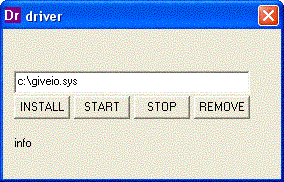
You need this header files
#include <winsvc.h> #include <winerror.h>
add this variables
SC_HANDLE hSCMan = NULL; char szPath[MAX_PATH], szDriver[MAX_PATH];
BOOL CNtdriverDlg::OnInitDialog() { CDialog::OnInitDialog(); SetIcon(m_hIcon, TRUE); // Set big icon SetIcon(m_hIcon, FALSE); // Set small icon // TODO: Add extra initialization here SetDlgItemText(IDC_DRVNAME,"c:\\giveio.sys"); // connect to local service control manager if ((hSCMan = OpenSCManager(NULL, NULL, SC_MANAGER_ALL_ACCESS)) == NULL) { AfxMessageBox( "Can't connect to service control manager",MB_OK); } return TRUE; } void CNtdriverDlg::OnClose() { if (hSCMan != NULL) CloseServiceHandle(hSCMan); CDialog::OnClose(); } //******************************************************** //******************************************************** void CNtdriverDlg::OnInstall() { DWORD dwStatus; GetServiceName( szPath, szDriver); dwStatus = DriverInstall(szPath, szDriver); DisplayStatus(dwStatus); } void CNtdriverDlg::OnStart() { DWORD dwStatus; GetServiceName(szPath, szDriver); dwStatus = DriverStart(szDriver); DisplayStatus( dwStatus); } void CNtdriverDlg::OnStop() { DWORD dwStatus; GetServiceName( szPath, szDriver); dwStatus = DriverStop(szDriver); DisplayStatus( dwStatus); } void CNtdriverDlg::OnRemove() { DWORD dwStatus; GetServiceName( szPath, szDriver); dwStatus = DriverRemove(szDriver); DisplayStatus( dwStatus); } //******************************************************************* //******************************************************************* void CNtdriverDlg::GetServiceName( LPSTR lpPath, LPSTR lpDriver) { GetDlgItemText(IDC_DRVNAME, lpPath, MAX_PATH); _splitpath(lpPath, NULL, NULL, lpDriver, NULL); } DWORD CNtdriverDlg::DriverInstall(LPSTR lpPath, LPSTR lpDriver) { BOOL dwStatus = TRUE; SC_HANDLE hService = NULL; // add to service control manager's database if ((hService = CreateService(hSCMan, lpDriver, lpDriver, SERVICE_ALL_ACCESS, SERVICE_KERNEL_DRIVER, SERVICE_DEMAND_START, SERVICE_ERROR_NORMAL, lpPath, NULL, NULL, NULL, NULL, NULL)) == NULL) dwStatus = GetLastError(); else CloseServiceHandle(hService); return dwStatus; } // DriverInstall DWORD CNtdriverDlg::DriverStart(LPSTR lpDriver) { BOOL dwStatus = TRUE; SC_HANDLE hService = NULL; // get a handle to the service if ((hService = OpenService(hSCMan, lpDriver, SERVICE_ALL_ACCESS)) != NULL) { // start the driver if (!StartService(hService, 0, NULL)) dwStatus = GetLastError(); } else dwStatus = GetLastError(); if (hService != NULL) CloseServiceHandle(hService); return dwStatus; } // DriverStart DWORD CNtdriverDlg::DriverStop(LPSTR lpDriver) { BOOL dwStatus = TRUE; SC_HANDLE hService = NULL; SERVICE_STATUS serviceStatus; // get a handle to the service if ((hService = OpenService(hSCMan, lpDriver, SERVICE_ALL_ACCESS)) != NULL) { // stop the driver if (!ControlService(hService, SERVICE_CONTROL_STOP, &serviceStatus)) dwStatus = GetLastError(); } else dwStatus = GetLastError(); if (hService != NULL) CloseServiceHandle(hService); return dwStatus; } // DriverStop DWORD CNtdriverDlg::DriverRemove(LPSTR lpDriver) { BOOL dwStatus = TRUE; SC_HANDLE hService = NULL; // get a handle to the service if ((hService = OpenService(hSCMan, lpDriver, SERVICE_ALL_ACCESS)) != NULL) { // remove the driver if (!DeleteService(hService)) dwStatus = GetLastError(); } else dwStatus = GetLastError(); if (hService != NULL) CloseServiceHandle(hService); return dwStatus; } // DriverRemove void CNtdriverDlg::DisplayStatus( DWORD dwStatus) { CString str;//char szStatus[128]; str.Format("%d",dwStatus); SetDlgItemText(IDC_STATUS, str); } // DisplayStatus